Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- tensorflow
- ubuntu18.04
- 웹페이지 기본
- raspberrypi
- 삼성SW역량테스트
- HTML
- html input
- 라즈베리파이
- jupyter
- 코딩테스트후기
- RIAD0
- TensorflowServer
- html태그정리
- RAID구축
- C언어
- multicast
- Raid
- 삼성sw역량테스트b형
- CSS
- html환경구축
- 개념설명
- Ubunrtu
- docker
- 다항회귀예제
- 다항회귀
- HTML예제
- RAID개념설명
- html input tag
- Linux
- 멀티캐스트
Archives
- Today
- Total
Easy ways
[C 언어] 프로그램이 read 에서 멈출때 해결법(read() 타임 아웃 설정:select) 본문
반응형
시스템을 구현 하다보면 read() 함수에서 대기상태에 빠져
코드가 가만히 멈춰있는 경우가 생길 수 있습니다.
이런경우 해결책으로 여러가지 방법이 있지만
저는 주로 read 함수에 타임아웃을 설정하여 일정 시간 수신을 기다리다가
아무것도 입력되지않으면 빠져나오는 방식을 사용합니다.
이러한 timeout 을 설정하는 방식도 다양하지만
오늘은 제가 가장 애용하는 방식인 select 함수를 통한 예제를 보여드리겠습니다.
int read_with_timeout(int fd, char *buf, int buf_size, int timeout_ms)
{
int rx_len = 0;
struct timeval timeout;
fd_set readFds;
// recive time out config
// Set 1ms timeout counter
timeout.tv_sec = 0;
timeout.tv_usec = timeout_ms*1000;
FD_ZERO(&readFds);
FD_SET(fd, &readFds);
select(fd+1, &readFds, NULL, NULL, &timeout);
if(FD_ISSET(fd, &readFds))
{
rx_len = read(fd, buf, buf_size);
}
return rx_len;
}
제가 자주 쓰는 함수 입니다.
이 함수는 파일디스크립터(fd)와 수신된 데이터를 저장할 버퍼 그리고 버퍼의 사이즈(*buf, bufsize),
몇초 후에 타임 아웃할 것인지에 대한 변수(timeout_ms) 를 미리초 단위로 입력 받습니다.
테스트 코드
위 코드가 제대로 작동하는지 테스트해보기 위한 예제 코드입니다.
간단한 udp 소켓을 하나 열어서 그 주소로 딱 한번의 패킷을 보내보겠습니다.
timeout은 1초로 주고 5초동안 기다리도록 하겠습니다.
#include <stdio.h>
#include <string.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/time.h>
#include <sys/select.h>
#include <fcntl.h> // Contains file controls like O_RDWR
#include <termios.h> // Contains POSIX terminal control definitions
#include <unistd.h> // write(), read(), close()
int read_with_timeout(int fd, char *buf, int buf_size, int timeout_ms)
{
int rx_len = 0;
struct timeval timeout;
fd_set readFds;
// recive time out config
// Set 1ms timeout counter
timeout.tv_sec = 0;
timeout.tv_usec = timeout_ms*1000;
FD_ZERO(&readFds);
FD_SET(fd, &readFds);
select(fd+1, &readFds, NULL, NULL, &timeout);
if(FD_ISSET(fd, &readFds))
{
rx_len = read(fd, buf, buf_size);
}
return rx_len;
}
int open_udp_socket( char *ip_addr,int port)
{
int reuse=1;
int rtn;
int sockfd;
struct sockaddr_in saddr;
sockfd = socket(PF_INET, SOCK_DGRAM, IPPROTO_UDP);
if (sockfd < 0)
{
return -1;
}
saddr.sin_family = AF_INET;
saddr.sin_port = htons(port);
saddr.sin_addr.s_addr = inet_addr(ip_addr);
if(setsockopt(sockfd, SOL_SOCKET, SO_REUSEADDR, (const char*)&reuse, sizeof(reuse)) < 0)
{
close(sockfd);
return -1;
}
rtn = bind( sockfd, (struct sockaddr *) &saddr, sizeof(saddr));
if (rtn < 0)
{
close(sockfd);
return -1;
}
return sockfd;
}
#define READ_BUF_SIZE 1024
int main(void)
{
char *ip = "192.168.0.20";
int port = 8200 ;
char rx_buf[READ_BUF_SIZE]={0,};
int fd, i, rx_len;
fd = open_udp_socket(ip,port);
for(i = 0; i < 5; i++)
{
// read timeout 1000ms = 1 sec
rx_len = read_with_timeout(fd,rx_buf,READ_BUF_SIZE,1000);
if(rx_len > 0 )
printf("recvied data(%d): %s\n",rx_len, rx_buf);
else
printf("nothing recived..!\n");
}
close(fd);
return 0 ;
}
Result
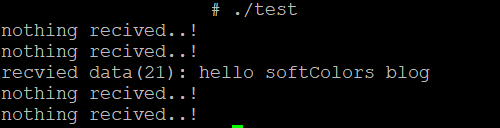
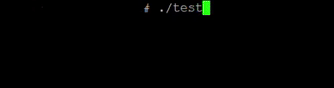
실제 흐름을 보여드리고 싶어 gif 파일로 변환하다 보니까 화질이 많이 깨졌네요
read 함수를 사용했음에도 기다리지 않고 1초마다 프로그램이 돌아가는 것을 볼 수 있습니다.
반응형
'프로그래밍 언어 > C언어' 카테고리의 다른 글
[Linux/C 언어] 멀티캐스트 송신 코드 및 테스트 (Multicast Sender) (3) | 2021.02.26 |
---|---|
[C 언어] 시리얼 통신 Open 함수 (struct termios) (1) | 2021.02.23 |
[C 언어] 디렉토리에서 가장 최근에/오래전에 수정된 파일 찾기. (0) | 2021.01.14 |
[C 언어] 현재 시간을 표시하는 방법 [time, ftime, ctime] (0) | 2021.01.13 |
Comments